Evolution Strategies (\(\mu,\lambda\)) (ES)¶
A module for the evolution strategies (\(\mu,\lambda\)) with adaptive strategy vectors.
Original paper: Bäck, T., Fogel, D. B., Michalewicz, Z. (Eds.). (2018). Evolutionary computation 1: Basic algorithms and operators. CRC press.
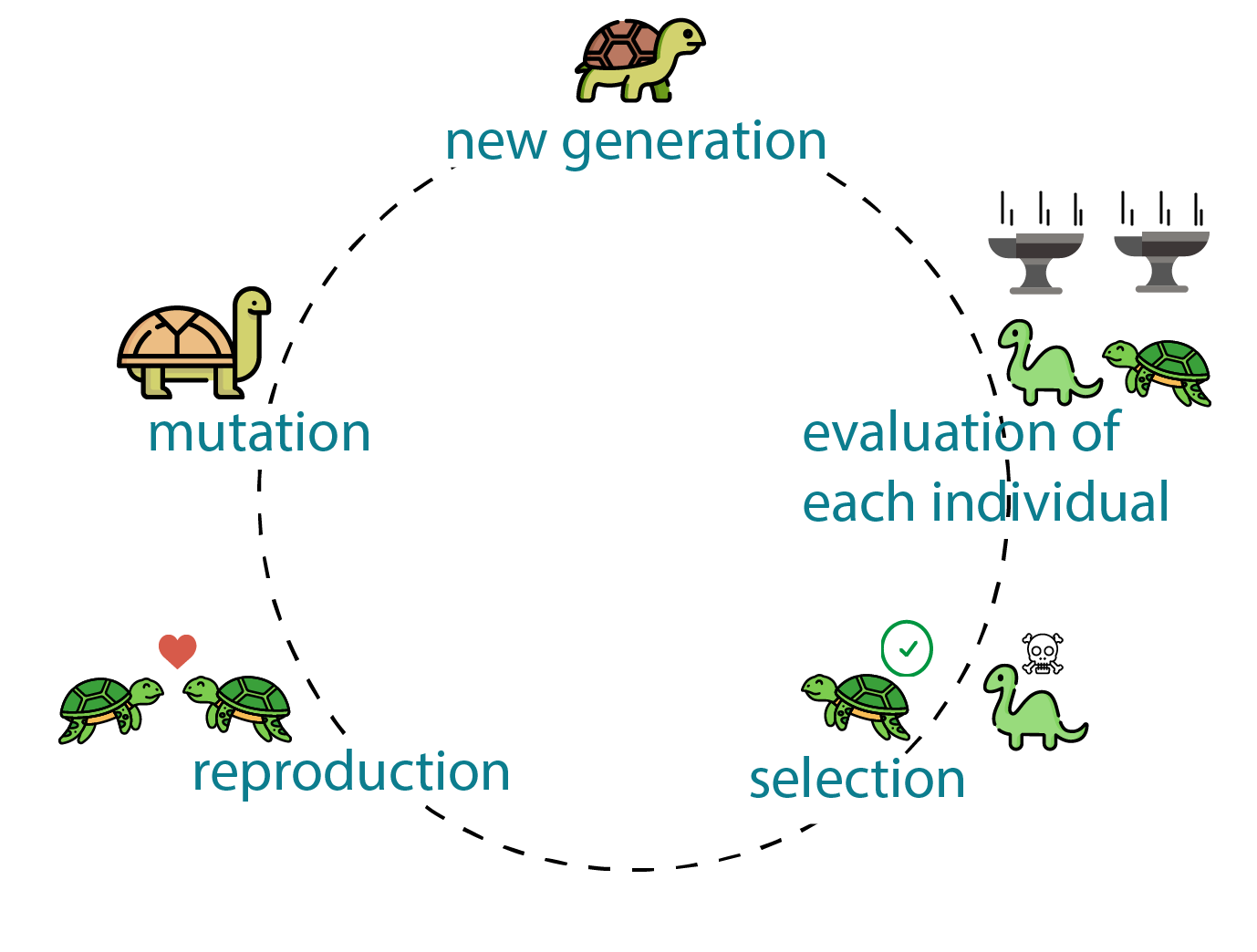
What can you use?¶
Multi processing: ✔️
Discrete spaces: ✔️
Continuous spaces: ✔️
Mixed Discrete/Continuous spaces: ✔️
Parameters¶
-
class
neorl.evolu.es.
ES
(mode, bounds, fit, lambda_=60, mu=30, cxmode='cx2point', alpha=0.5, cxpb=0.6, mutpb=0.3, smin=0.01, smax=0.5, clip=True, ncores=1, seed=None, **kwargs)[source]¶ Parallel Evolution Strategies
- Parameters
mode – (str) problem type, either
min
for minimization problem ormax
for maximizationbounds – (dict) input parameter type and lower/upper bounds in dictionary form. Example:
bounds={'x1': ['int', 1, 4], 'x2': ['float', 0.1, 0.8], 'x3': ['float', 2.2, 6.2]}
lambda_ – (int) total number of individuals in the population
mu – (int): number of individuals to survive to the next generation, mu < lambda_
cxmode – (str): the crossover mode, either ‘cx2point’ or ‘blend’
alpha – (float) Extent of the blending between [0,1], the blend crossover randomly selects a child in the range [x1-alpha(x2-x1), x2+alpha(x2-x1)] (Only used for cxmode=’blend’)
cxpb – (float) population crossover probability between [0,1]
mutpb – (float) population mutation probability between [0,1]
smin – (float): minimum bound for the strategy vector
smax – (float): maximum bound for the strategy vector
ncores – (int) number of parallel processors
seed – (int) random seed for sampling
-
evolute
(ngen, x0=None, verbose=False)[source]¶ This function evolutes the ES algorithm for number of generations.
- Parameters
ngen – (int) number of generations to evolute
x0 – (list of lists) the initial position of the swarm particles
verbose – (bool) print statistics to screen
- Returns
(tuple) (best individual, best fitness, and a list of fitness history)
Example¶
from neorl import ES
#Define the fitness function
def FIT(individual):
"""Sphere test objective function.
F(x) = sum_{i=1}^d xi^2
d=1,2,3,...
Range: [-100,100]
Minima: 0
"""
check=all([item >= BOUNDS['x'+str(i+1)][1] for i,item in enumerate(individual)]) and all([item <= BOUNDS['x'+str(i+1)][2] for i,item in enumerate(individual)])
if not check:
raise Exception ('--error check fails')
y=sum(x**2 for x in individual)
return y
#Setup the parameter space (d=5)
nx=5
BOUNDS={}
for i in range(1,nx+1):
BOUNDS['x'+str(i)]=['float', -100, 100]
es=ES(mode='min', bounds=BOUNDS, fit=FIT, lambda_=80, mu=40, mutpb=0.1,
cxmode='blend', cxpb=0.7, ncores=1, seed=1)
x_best, y_best, es_hist=es.evolute(ngen=5, verbose=1)
Notes¶
Too large population mutation rate
mutpb
could destroy the population, the recommended range for this variable is between 0.01-0.4.Too large
smax
will allow the individual to be perturbed in a large rate.Too small
cxpb
andmutpb
reduce ES exploration, and increase the likelihood of falling in a local optima.Usually, population size
lambda_
between 60-100 shows good performance along withmu=0.5*lambda_
.Look for an optimal balance between
lambda_
andngen
, it is recommended to minimize population size to allow for more generations.Total number of cost evaluations for ES is
lambda_
*(ngen + 1)
.cxmode='blend'
withalpha=0.5
may perform better thancxmode='cx2point'
.